
Different Ways To Declare Functions in Javascript
Thu Mar 28 2024
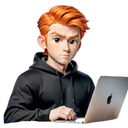
Eric Thomas D. Cabigting
Function Declaration
- Using the `function` keyword
- These functions are hoisted on top of the scope where you defined them. Which enables you to use the functions even if they are declared at the end of your code.
function foo(bar) {
return bar-1;
}
Function Expressions
- Also using the `function` keyword
- You are assigning it to a `variable` that can be use later on
- Difference of Expressions vs Declarations is that the Function Expressions are not hoisted to the top of the global scope
const myValue = function myFunction(foo,bar) {
return bar * foo;
}
Another variation of this is a nameless function directly assigned to a variable like below:
const addition = function(val1,val2){
return val1 + val2;
}
Functions Expressions in Objects
- Assigning a nameless functions in a property of an object
const mdas = {
mul: function(val1,val2) {return val1 * val2;},
div: function(val1,val2) {return val1 / val2;},
add: function(val1,val2) {return val1 + val2;},
sub: function(val1,val2) {return val1 - val2;},
}
Named Functions Expressions
https://www.youtube.com/watch?v=4U0Snxxej74&t=207s
Let's Connect
Hey! My inbox is always free! Currently looking for new opportunities. Email me even just to say Hi! or if you have questions! I will get back to you as soon as possible!