
Keeping It this Together: A Closure Look at State Management and Factory Functions
Fri Feb 28 2025
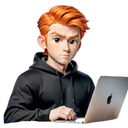
Eric Thomas D. Cabigting
And no, Im not talking about factories closing with the most recent lay-offs. Instead lets talk about `this`, closure, state management and factory functions in javascript.
`This` what is it? How does it work?
Lets start with `this`. In JavaScript, the this keyword refers to the current execution context of a function. It can be a bit tricky to understand because its value can change depending on how a function is called. Here are some basic rules to determing the value of this:
- Global Context:
- In the global context (outside of any function or object), `this` refers to the global object.
- In browsers the global object is `windows`.
- In Node.js, the global object is `global`.
- In the global context (outside of any function or object), `this` refers to the global object.
console.log(this); // Executing this code in a Browser, returns `window`, while running it in node.js in a server returns `global`
- Function Context:
- Inside a regular function (not an arrow function), the value of `this` depends on how the function is called:
- If the function is called in the global context, `this` refers to the global object (or `undefined` in strict mode).
- If the function is a method of an object, `this` referes to the object that the function is a method of.
- Inside a regular function (not an arrow function), the value of `this` depends on how the function is called:
function sampleFunction() {
console.log(this);
}
sampleFunction(); // In non-strict mode: `window` (browser) or `global` (Node.js)
// In strict mode: `undefined`
const myObj = {
method: sampleFunction
};
myObj.method(); // `this` refers to `myObj`
- Method Context:
- When a function is called as a method of an object, `this` referes to the object that the method belongs to.
const myObj = {
name: "Alice",
greet: function() {
console.log(`Hello, ${this.name}`);
}
};
myObj.greet(); // `this` refers to `myObj`; outputs: "Hello, Alice"
- Constructor context:
- When a function is used as a constructor (with the `new` keword), `this` refers to the new object being created.
function Person(name) {
this.name = name;
}
const alice = new Person("Alice");
console.log(alice.name); // `this` refers to the new object; outputs: "Alice"
- Arrow Functions:
- Arrow functions do not have their own `this` (makes sense since arrow functions are anonymouse functions). Instead, they inherit `this` from the enclosing lexical context (the context where the arrow function is defined).
const obj = {
name: "Alice",
greet: () => {
console.log(`Hello, ${this.name}`);
}
};
obj.greet(); // `this` refers to the global object (or `undefined` in strict mode)
- Explicit Binding with `bind`,`call`, and `apply`:
- This `bind`,`call`, and `apply` methods can be used to explicitly set the value of `this` for a function.
- `bind`: Creates a new function with this bound to the specific object
- `call` and `apply`: Immediately invokes a function with `this` set to a specific object.
- This `bind`,`call`, and `apply` methods can be used to explicitly set the value of `this` for a function.
function greet() {
console.log(`Hello, ${this.name}`);
}
const alice = { name: "Alice" };
const bob = { name: "Bob" };
greet.call(alice); // `this` refers to `alice`; outputs: "Hello, Alice"
greet.apply(bob); // `this` refers to `bob`; outputs: "Hello, Bob"
const greetAlice = greet.bind(alice);
greetAlice(); // `this` refers to `alice`; outputs: "Hello, Alice"
State Management for your everyday coding problems.
State management in JavaScript refers to the process of managing and controlling the data (state) that determines the behavior and rendering of an application. It involves storing, updating, and sharing data across different parts of an application in a predictable and efficient way. State management is crucial for building complex applications, especially those with dynamic user interfaces, such as single-page applications (SPAs).
What is State?
State is the data that changes over time and influences the behavior or appearance of an application. Examples of state include:
- User input (e.g., form data, search queries).
- UI state (e.g., whether a modal is open or closed).
- Data fetched from an API (e.g., a list of products or user profiles).
- Authentication status (e.g., whether a user is logged in).
Statemanagement Techniques in Javascript:
- Local State
- State is manage within a function or block scope, see code example:
function counter() {
let count = 0; // Local state
return {
increment: function() {
count++;
console.log("Count:", count);
},
decrement: function() {
count--;
console.log("Count:", count);
},
getCount: function() {
return count;
}
};
}
const myCounter = counter();
myCounter.increment(); // Count: 1
myCounter.increment(); // Count: 2
myCounter.decrement(); // Count: 1
console.log(myCounter.getCount()); // 1
- Closures
- Encapsulate state and expose methods to interact with it.
- Closures allow you to encapsulate state and expose only the necessary methods to interact with it.
function createState(initialState) {
let state = initialState; // Encapsulated state
return {
getState: function() {
return state;
},
setState: function(newState) {
state = { ...state, ...newState }; // Immutable update
console.log("State updated:", state);
}
};
}
const appState = createState({ count: 0, user: "Guest" });
appState.setState({ count: 1 }); // State updated: { count: 1, user: "Guest" }
appState.setState({ user: "Alice" }); // State updated: { count: 1, user: "Alice" }
console.log(appState.getState()); // { count: 1, user: "Alice" }
- Event-Driven
- Use events to notify components of state changes.
- You can use event listeners to update and react to state changes.
const state = { count: 0 };
function updateState(newState) {
Object.assign(state, newState); // Update state
console.log("State changed:", state);
// Trigger a custom event
const event = new CustomEvent("stateChange", { detail: state });
document.dispatchEvent(event);
}
// Listen for state changes
document.addEventListener("stateChange", (event) => {
console.log("State updated to:", event.detail);
});
// Update state
updateState({ count: 1 }); // State changed: { count: 1 }
updateState({ count: 2 }); // State changed: { count: 2 }
- State Management with a Pub/Sub Pattern
- The Publish/Subscribe (Pub/Sub) pattern allows components to subscribe to state changes and react accordingly.
function createPubSub() {
const subscribers = [];
return {
subscribe: function(callback) {
subscribers.push(callback);
},
publish: function(data) {
subscribers.forEach((callback) => callback(data));
}
};
}
const pubSub = createPubSub();
// Subscribe to state changes
pubSub.subscribe((state) => {
console.log("State updated:", state);
});
// Update state and notify subscribers
let state = { count: 0 };
function setState(newState) {
state = { ...state, ...newState }; // Immutable update
pubSub.publish(state);
}
setState({ count: 1 }); // State updated: { count: 1 }
setState({ count: 2 }); // State updated: { count: 2 }
** Immutable update: means that instead of modifying the existing state directly, you create a new copy of the state with the desired changes. This ensures that the original state remains unchanged, which is a key principle in functional programming and helps avoid unintended side effects.
- State Management with a Factory Function
- A factory function can create multiple instances of stateful objects.
function createCounter(initialValue = 0) {
let count = initialValue; // Encapsulated state
return {
increment: function() {
count++;
console.log("Count:", count);
},
decrement: function() {
count--;
console.log("Count:", count);
},
getCount: function() {
return count;
}
};
}
const counterA = createCounter();
const counterB = createCounter(10);
counterA.increment(); // Count: 1
counterB.decrement(); // Count: 9
console.log(counterA.getCount()); // 1
console.log(counterB.getCount()); // 9
- State Management with a Proxy
- The Proxy object can be used to intercept and manage state changes. Check out the mdn web docs here for more details.
const state = { count: 0 };
const stateProxy = new Proxy(state, {
set: function(target, property, value) {
console.log(`State changed: ${property} = ${value}`);
target[property] = value; // Update state
return true;
}
});
stateProxy.count = 1; // State changed: count = 1
stateProxy.count = 2; // State changed: count = 2
console.log(state); // { count: 2 }
Closure, do you get it?
A closure is a function that remembers the variables from its outer scope even after the outer function has finished execution. It is created when an inner function retains access to variables from its outer (enclosing) function.
- What are Closures useful for?
- Data Encapsulation (keeping variable private)
- Function Factories (creating specialize functions). Check above for other examples
- Memoization and Caching (storing computed values)
Here are some code examples of Closure's usefulness:
- Data Encapsulation
- Used in real-world scenarios like private counters, API rate limits, and security.
function createCounter() {
let count = 0; // Private variable
return function () {
count++;
return count;
};
}
const counter = createCounter();
console.log(counter()); // 1
console.log(counter()); // 2
console.log(counter()); // 3
Here, count persists because of the closure, but it’s not directly accessible outside createCounter().
- Function Factory
- Used in configurable event handlers, UI components, and role-based access controls.
function createGreeting(greeting) {
return function (name) {
return `${greeting}, ${name}!`;
};
}
const sayHello = createGreeting("Hello");
console.log(sayHello("Alice")); // "Hello, Alice!"
console.log(sayHello("Bob")); // "Hello, Bob!"
createGreeting("Hello")remembers the "Hello" greeting, so we don’t need to pass it every time.
- Memoization
- Used in caching API results, expensive calculations, and improving performance.
function memoizedAdder() {
let cache = {}; // Stores previous results
return function (num) {
if (num in cache) {
console.log("Fetching from cache...");
return cache[num];
}
console.log("Calculating...");
cache[num] = num + 10;
return cache[num];
};
}
const add10 = memoizedAdder();
console.log(add10(5)); // "Calculating..." → 15
console.log(add10(5)); // "Fetching from cache..." → 15
The closure remembers previous results and avoids redundant calculations.
Lets get into Factory
A factory function is a design pattern in JavaScript where a function is used to create and return objects. Instead of using new with a constructor function (as in classical object-oriented programming), you call the factory function directly, and it returns a new object.
- How to Identify a Factory Function
- No `new` keyword
- Unlike constuctor functions, factory functions dont require the `new` keyword to create objects.
- Encapsulation
- Factory functions can encapsulate private state and explose only necessary methods or properties.
- Flexibility
- They allow you to create objects with different configurations or behaviors based on the input paramaters.
- No `this` binding
- Factory functions don't rely on `this`, making them simpler and less error-prone in certain scenarios.
- No `new` keyword
Check out this code example of a factory function that create user objects:
function createUser(name, age) {
// Private state (encapsulated)
let isLoggedIn = false;
// Return an object with methods and properties
return {
name,
age,
login() {
isLoggedIn = true;
console.log(`${this.name} has logged in.`);
},
logout() {
isLoggedIn = false;
console.log(`${this.name} has logged out.`);
},
getStatus() {
return `${this.name} is ${isLoggedIn ? "logged in" : "logged out"}.`;
}
};
}
// Create users using the factory function
const alice = createUser("Alice", 25);
const bob = createUser("Bob", 30);
alice.login(); // Alice has logged in.
console.log(alice.getStatus()); // Alice is logged in.
bob.logout(); // Bob has logged out.
console.log(bob.getStatus()); // Bob is logged out.
We explored how closures, factory functions, and the this keyword work together to create flexible and maintainable state management solutions. By leveraging these concepts, we can encapsulate data, preserve state across function executions, and structure reusable object-like behavior. Understanding these principles not only enhances code organization but also provides a deeper insight into JavaScript’s powerful function-based design patterns. Whether managing counters, handling UI components, or optimizing performance, mastering these techniques empowers developers to write cleaner, more efficient code.
Let's Connect
Hey! My inbox is always free! Currently looking for new opportunities. Email me even just to say Hi! or if you have questions! I will get back to you as soon as possible!