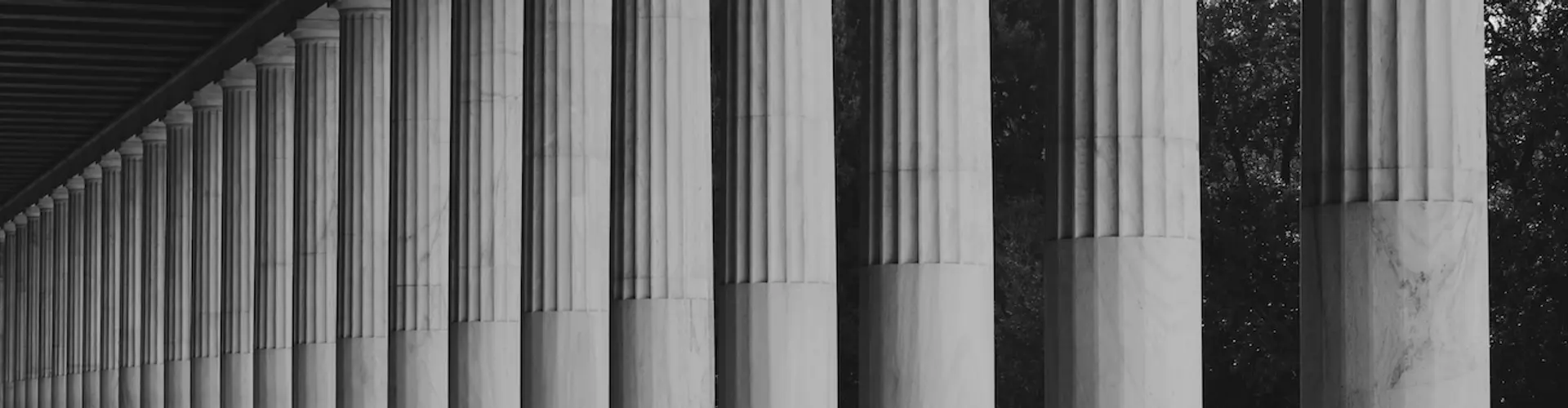
Object Oriented Programming - The Pillars
Thu Feb 17 2022
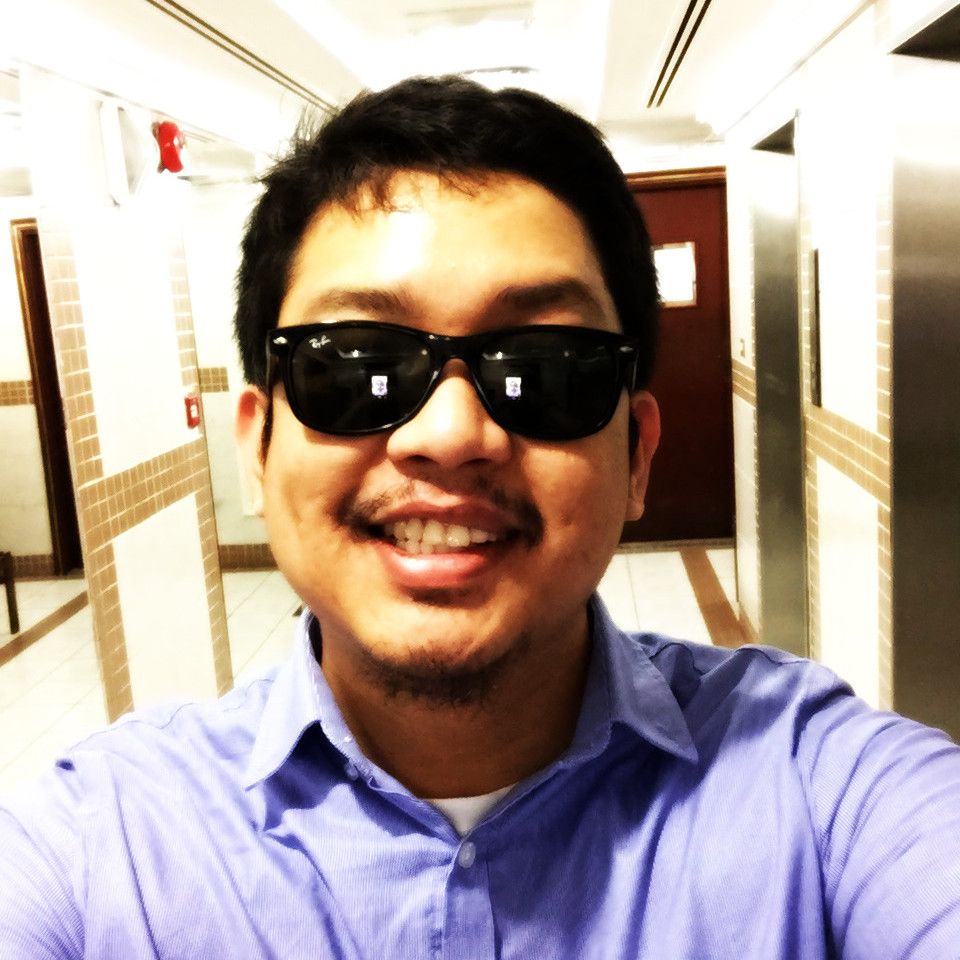
Eric Thomas D. Cabigting
Abstraction
From docs.microsoft.com
Abstraction Modeling the relevant attributes and
interactions of entities as classes to define
an abstract representation of a system.
On a more basic definition, Abstraction in OOP is to generalize all characteristics of a real world object into a single representation. For example, if you have a phone, a laptop, and a smart watch, we can all abstract these into a device. From this the abstract class device, can expose only the necessary class members like device wieght, device brand, device screen size. The user does not need to know how this devices connects to the internet. Or how it connects to the cellular network. If you are in a coffeshop, you can see over the counter they are selling coffee, cookies, bread, fruit cups, and a sandwhich. From a point of sales perspective, we can abstract these as products. In the point of sale point of view, the products exposes the following properties, the price, the size, the amount of tax. It does not need to know how we compute the tax.
In C#, Abstraction is possible by declaring a classes using the abstract keyword, the following are the characteristics of an abstract class in c#:
- Class that inherits from an abstract class must implement all the abstracts members.
- From my example above products and device classes are the base classes from which classes inherits from.
- Abstract classes in C# cannot be use to instantiate an object. They can only be inherited from.
- Properties and methods of an abstract class can be define as abstract as well.
Encapsulation
From docs.microsoft.com
Encapsulation Hiding the internal state and functionality
of an object and only allowing access through a public
set of functions.
From the description above, we can say that the process of encapsulation preventing access to implementation details from the object. It also allows us to define the access level of the class. So basically we are prevented from accidentally changing the data from outside the class.
In C# you are can use encapsulation with the following access modifiers:
- Public - any public member variable, function, or methods have no restrictions and are accessible outside of the class.
- Private - only allow member variable, functions or method to be access from inside the class or through an instantiated object.
- Protected - only child classes and the current class can access its members.
- Internal - exposes class members to other functions and objects in the current assembly
- Protected Internal - allows access to only child classes within the same application.
- Private Protected - cannot be access in the entire program, can be access from the containing class, not accessible in the current assembly, cannot be access by the subclass, but can be access by the subclass on the current assembly.
Inheritance
From docs.microsoft.com
Inheritance Ability to create new abstractions based
on existing abstractions.
Among all the pillars of OOP this is my favorite. When you abstract a real life object. You can create the generalize members of the class and have this inherited to child classes that are similar. This promotes Reusability.
In c# Inheritance is done by using a : (colon) on the declartion of a class. See the example below:
public abstract class Vehicle
{
public int Length {get;set;}
public string TypeOfFuel {get;set;}
public string EngineName {get;set;}
public int Height {get;set;}
}
public class Airplane : Vehicle
{
public int WingSpan {get;set;}
public int FlightHours {get;set;}
public string FlightNumber {get;set;}
}
public class Boat : Vehicle
{
public int NumberOfSails {get;set;}
public string RudderType {get;set;}
public string HullType {get;set;}
}
From the example above we have the Boat and Airplane class inherits from the vehicle parent class. This means boat and airplan gets all the members of the vehicle class. So we can say vehicle is the Super Class and both the airplane and boats are our Sub Classes.
Polymorphism
From docs.microsoft.com
Polymorphism Ability to implement inherited properties
or methods in different ways across multiple abstractions.
In C# Polymorphism is commonly done via Method Overloading. You can implement multiple function of the same name in the same class. The functions must have different method signatures, for example number of parameters, the data type of parameters, and the order of the parameters. This type of polymorpism is called Compile Time Polymorphism. See example below:
public class Car
{
void StartEngine(KeyPosition TurnKey)
{
// start engine
}
void StartEngine(bool ButtonPress)
{
// start engine
}
enum KeyPosition
{
Off=1,
On=2,
Start=3
}
}
Another type of Polymorphism in c# is called Run-Time Polymorphism. This occurs when an object is bound with the functionality at runtime. This is achived via Method Overriding. This feature allows a subclass or child class to have its own implementation of a method that is already given from the super class or parent class. The method in the subclass that has the same name, number or arguments, and type of arguments as the one in the parent class, then it is to override the method in the superclass.
The following keywords are available in c# to perform method overriding:
- virtual - this is use in the base class. It spefices that the method can be overriden in the derived class or the subclass.
- override - this is use in the subclass or derived class. It is use to modify the virtual class from the base class.
- base - this is used to access members of the base class from within the sub or derived class. It cannot be use within a static method. Basically it is use to access the constructor methods and functions of the base class. It specifies which constructor of the base class should be invoke when creating the instance of the subclass.
Example using virtual and override:
class Vehicle
{
public virtual void SoundHorn()
{
Console.WriteLine("Vehicle Horn sound!");
}
}
public Car : Vehicle
{
public override void SoundHorn()
{
Console.WriteLine("Beep Beep!");
}
}
public Truck : Vehicle
{
public override void SoundHorn()
{
Console.WriteLine("Boom boom!!");
}
}
Example using base keyword:
class Vehicle
{
string Color = "Black";
public virtual void SetDefaultColor()
{
Color = "Red";
Console.WriteLine("Vehicle Default color: " + Color);
}
}
class Car : Vehicle
{
string CarColor = "White"
public void SetCarColor()
{
base.SetDefaultColor();
Console.WriteLine("Car color: " + CarColor);
}
}
Let's Connect
Hey! My inbox is always free! Currently looking for new opportunities. Email me even just to say Hi! or if you have questions! I will get back to you as soon as possible!