
The best approach on solving Leet Code problems
Tue Mar 04 2025
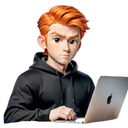
Eric Thomas D. Cabigting
I have been solving LeetCode problems on my spare time for a while now. There are problems that takes me a few minutes to solve, some takes me hours, even days(dont worry I take breaks in between). My goal is to improve my problem solving skills while also getting familiar with syntax and language features. Currently I focus more on Javascript that any other language(Go, C#, C++).
The questions in LeetCode are grouped by Difficulty, namely easy, medium, hard. Often than not, I feel like a hard question should have been easy, or a medium should have been hard and the inverse. During this time, I was able to formulate somewhat an approach or a way of thinking if you will on solving this problems.
Here is how I approach LeetCode problems thats seems to work for me.
Understand the Problem
LeetCode problems are somewhat straight forward and have very good explanations. They break down the problem for people to not have different understand, but rather having all problem solvers arrive the same conclusion. If you are like me. But that doesnt mean the problem can be absorbed and understood in one reading. My approach here is with the following points:
- What is the input?
- I try to thoroughly understand the inputs provided for the problem, identify the datatypes(numbers, string, booleans, arrays, etc) provided in all given test cases.
- Finding and understanding the constraints. Do we have input size limit, what are the edge cases for the input? Are empty inputs possible? Do we have min/max values? What about special characters? These are some of the points I try to check when I analyze the input for the problem
- What is the output?
- What is the expected output? Do we return a number, an array or an object? Is the expected output in a certain format? Is it a single value or a collection of values?
- Consider edge cases. With the given input, is it possible that the output is null or undefined? These are some important question to help you understand the problem.
- What are the requirements and rules we must follow?
- Are there any specific conditions or edge cases we need to consider?
- What transformations or operations need to be performed on the input produce the expected output?
- While looking for the requirements, always take a step back every now and then to see how the bigger picture is forming with each rules/requirements being describe in the problem
Work Through the Examples(test Cases)
LeetCode made sure to give concrete and concise test cases when you are trying to test your solution. Although not all were shown when you try to submit. These initial test cases are great way into further understanding the problem. Test cases provides the expected input type wether it is of different data types, for each cases or a single type for the whole problem. Stop for a few seconds and see the pattern forming with all the inputs required for each cases. Give it a second, see all the inputs. You will see a pattern. That pattern more often than not is the actual logic/key to solving the problem.
Here is are some example of inputs when trying to write groupBy function:
- Input: [1, 2, 3, 4, 5], grouping function: (num) => num % 2 === 0 ? 'even' : 'odd'
- Output: { odd: [1, 3, 5], even: [2, 4] }
- Input: ['apple', 'banana', 'cherry'], grouping function: (word) => word.length
- Output: { 5: ['apple'], 6: ['banana', 'cherry'] }
Did you find that sort of pattern to help you solve the problem?
If you look closely on the functions, they are returning a single value. And since the problem is to write a groupBy function, we need a 'group' of some sort. The return value can be our 'group' or key!
Break the Problem into smaller steps
Break down the problem into smaller, manageable tasks. And ask the following questions:
- What are the key steps needed to transform the input into the output
- What data structures or algorithms might be useful
Considering our previous example of writing a groupBy function, here are some points on how to breakdown the problem:
- Create an object to store the groups
- Loop through each element in the array
- For each element, figure out its expected group base on the groupingFunction provided.
- Return the grouped object
Go back to each step and break them down further, for example, in the third point above: For each element, figuour out its expected group base on the groupingFunction provided. We can break this down further by analysing the grouping function from the examples. We can see that in the examples we can see that the grouping functions always return a group or key. We can use this to determine the groups of each element.
Plan your Solution
This might be the most obvious and simple among the steps. You need to outline your solution first in pseudocode. This helps you foccus on the logic without getting slowed down by syntax errors. From there you can start tweaking the pseudocode with langauge functions or syntax to properly formulate the final solution to the problem.
With our groupBy function example, here is a pseudo code to the planned solution:
function groupBy(array, groupingFunction):
result = {}
for each element in array:
key = groupingFunction(element)
if key is not in result:
result[key] = []
result[key].push(element)
return result
Translate Pseudocode into Code
Once you have a more planned out and cleaned pseudocode, translate it into code. Focus on one step at a time and test as you go. This means, each line of the psuedocode, you go line per line into translating it into programming language code.
Given our example of groupBy function, here is the translated code into Javascript:
function groupBy(array, groupingFunction) {
const result = {};
for (const element of array) {
const key = groupingFunction(element);
if (!result[key]) {
result[key] = [];
}
result[key].push(element);
}
return result;
}
Testing your solution by Trial and Error:
Test your code with the examples you worked through earlier. Make sure it handles edge cases and unexpected inputs. This can be achieve by running your codde with different inputs. You might encouter errors on the first few runs. This is a good thing as it helps you simulate more scenarios and possible inputs.
Some test cases for our groupBy function:
- Input: [1, 2, 3, 4, 5], grouping function: (num) => num % 2 === 0 ? 'even' : 'odd'
- Expected Output: { odd: [1, 3, 5], even: [2, 4] }
- Input: ['apple', 'banana', 'cherry'], grouping function: (word) => word.length
- Expected Output: { 5: ['apple'], 6: ['banana', 'cherry'] }
- Edge Case: Empty array []
- Expected Output: {}
Optimizing, Refactoring, and Improving your Coden
Now that you have a working solution. You can do more by thinking about the following points:
- Time Complexity: Can you make it faster?
- Space Complexity: Can you reduce memory usage?
- Readability: Can you make the code cleaner or more concise?
On our groupBy function, the current solution is already optimize with a space complexity of 0(n) (where n is the size of the array) and time complexity of 0(n)
By following these a points, you can approach any leetcode problem systematically and solve them easily with given enough practice. You should put into practice these points that it become second nature to you
Let's Connect
Hey! My inbox is always free! Currently looking for new opportunities. Email me even just to say Hi! or if you have questions! I will get back to you as soon as possible!